Introduction
Hey there, coding aficionados! If you’ve ever wondered how your computer takes the code you’ve written and transforms it into something the machine can understand, you’re in for a treat. Let’s talk about compilers—the unsung heroes of the software development world. They are the magical bridges that turn human-readable code into something machines can actually execute. Whether you’re a beginner or a seasoned programmer, understanding how compilers work can totally level up your coding game. Ready to explore? Let’s dive in!
In this post, we’re going to break down the complex world of compiler construction, unraveling how this crucial process works from start to finish. Think of a compiler as a translator, taking your elegant, high-level code and transforming it into a language your computer understands: machine code. Trust me, the behind-the-scenes work is more exciting than you might think. So, let’s put on our coding hats and uncover the mystery of how compilers work their magic!
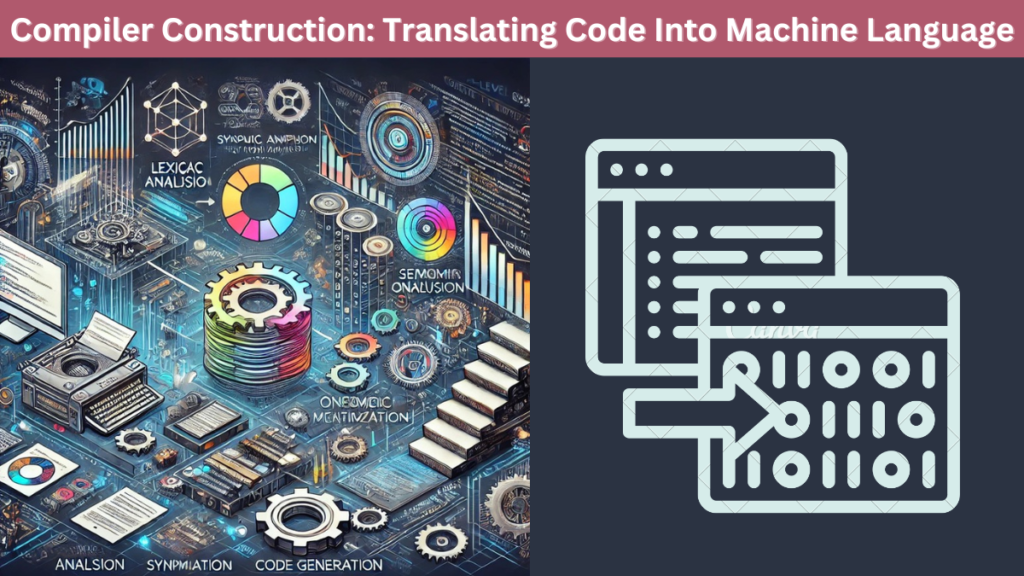
What is a Compiler?
Let’s start at the very beginning: What exactly is a compiler? In simple terms, a compiler is a program that translates code written in one programming language into machine language or bytecode. It’s like having a personal translator who speaks both your language (high-level code) and the machine’s language (binary or machine code). Without compilers, all our beautifully crafted programs would be nothing but gibberish to the computer.
But here’s the kicker—compilers don’t just translate code; they analyze and optimize it along the way. The translation process isn’t just about converting words; it involves checking for errors, ensuring that the code is syntactically correct, and even optimizing the code to run faster. Imagine getting your homework graded and corrected while it’s being translated into another language—now that’s some serious multitasking! So, the next time your code runs smoothly, thank a compiler for doing all the heavy lifting behind the scenes.
Overview of the Compilation Process
Now, let’s talk about how this compiling thing actually happens. It’s not a one-step process. Oh no! It involves several key stages, each of which plays an essential role in ensuring your code runs like a well-oiled machine. These stages include lexical analysis, syntax analysis, semantic analysis, optimization, and code generation. Each phase takes your code further along the path to becoming executable.
Let’s break it down step by step! First up, we have lexical analysis, where the compiler scans your code and splits it into “tokens”—the smallest units of meaning. Next, the syntax analysis checks if these tokens follow the rules of the programming language’s grammar, essentially parsing your code. Then comes semantic analysis, where the compiler ensures that your code makes sense logically (are you trying to add a number to a string? Not allowed!). Afterward, the code goes through optimization, where unnecessary operations are trimmed out, and finally, code generation happens, where the compiler creates machine-level instructions. If this sounds like a lot of moving parts—it is! But it all comes together beautifully at the end.
Lexical Analysis: Breaking Down Code Into Tokens
Let’s kick things off with lexical analysis. In this phase, the compiler takes your source code and breaks it down into manageable chunks, or tokens. These tokens can represent keywords, variables, operators, symbols, or other elements that make up the code. Think of this phase as the first step in making sense of your code—it’s like sorting your laundry into different piles: one for shirts, one for socks, and one for towels.
Once the code is broken down into tokens, the compiler can begin to understand what each piece represents. For example, it can identify a keyword like if
, or a variable like x
, or an operator like +
. This is a crucial step because it helps the compiler figure out how to proceed with further analysis. So, next time you see your code working smoothly, remember it all started with this careful breakdown of tokens!
Syntax Analysis: Ensuring Code Follows Grammar Rules
Once we have our tokens, it’s time for syntax analysis, the phase where the compiler checks if the code follows the proper grammar of the programming language. Much like how we have to follow rules when writing a sentence—like subject-verb agreement or punctuation—your code also needs to follow specific rules for it to make sense.
In this stage, the compiler takes those tokens and arranges them into a structure called an Abstract Syntax Tree (AST). This tree represents the grammatical structure of the code and shows how the different components are related. If something’s out of place, like a missing semicolon or a mismatched parentheses, the compiler will catch it right here. It’s like having an editor going through your work, making sure everything’s in the right place and free from awkward errors. Without this step, your code would be nothing but a confusing jumble!
Semantic Analysis: Checking for Meaning
Now that we have a structure in place, it’s time for semantic analysis, where the compiler checks the logic and meaning behind your code. This is where the real “thinking” happens. Just because the syntax is correct doesn’t mean the code is valid or meaningful. For example, if you’re trying to divide a string by a number, that’s an operation that doesn’t make any sense!
Semantic analysis ensures that all the operations in your code are logically sound. It also checks for things like variable declarations and type matching—whether the variable x
is assigned an integer when it should be, or if the data types align correctly. Think of this stage as the brain of the compiler, making sure everything you’ve written makes logical sense. Without this phase, you might end up with code that “works” on the surface but fails in a million other subtle ways!
Optimization: Making Code Faster
After the code passes semantic analysis, it moves on to the optimization stage. Here’s where the magic happens! Optimizing code means making it run faster, use less memory, or take fewer resources while maintaining the same functionality. It’s like getting a high-performance sports car that’s sleek, fast, and efficient.
There are many types of optimization that can take place here. You might see things like loop unrolling, where loops are simplified to reduce overhead, or dead code elimination, where unnecessary code is removed altogether. Optimization can be tricky, though—too much optimization can lead to complex code that’s harder to maintain. It’s a delicate balance, but when done right, optimization makes your program not only run better but also stand the test of time.
Code Generation: Translating to Machine Code
Next up is the code generation phase, where the compiler turns your beautiful code into machine language—the binary instructions that your computer can execute. This phase is like translating your recipe into a specific set of actions that the robot can perform to bake a cake. It’s the step that makes everything real. Without it, all the prior steps would just be intellectual exercises.
In this stage, the compiler generates assembly code or machine code that the computer’s processor can understand. This is the back end of the compiler, where things start to get really “technical.” If you’ve ever wondered how high-level code written in languages like Python, Java, or C++ is translated into something the computer can actually execute, this is the stage that does the job. And let me tell you, it’s a fascinating process!
Error Handling in Compilers
Of course, even the best compilers run into issues sometimes. Enter error handling. This phase is essential for making sure your code doesn’t break the whole process. If the compiler hits a snag—say, a syntax error or something illogical—it needs to handle it gracefully without just crashing. Think of it like a good friend who gently points out your mistakes rather than making you feel bad about them.
There are several ways compilers handle errors, like panic-mode recovery, where they simply skip over the error and continue parsing, or more sophisticated strategies like backtracking to try to fix it. The goal is to keep things moving forward, providing feedback so you can fix your code and keep going without too much disruption.
Conclusion: The Power of Compilers
And there you have it—compilers in a nutshell! They’re the unsung heroes that make it possible for our code to do what we want it to do. From lexical analysis all the way to code generation, each step of the compilation process is crucial for translating high-level, human-readable code into machine-understandable instructions.
Understanding compilers not only helps you write better, more efficient code, but it also opens up a whole new world of programming possibilities. So, whether you’re a coding newbie or a seasoned pro, remember that the next time you hit “run” on your code, there’s a whole complex process happening in the background that makes it all come to life. Keep coding, keep learning, and never stop exploring the magic of compilers!